The Shell
Depending on your operating system and C++ development environment used, there are different methods of starting a program. All of these methods ultimately rely on the operating system's facility to load and run a program.
While you can write C++ code which calls these facilities directly, your IDE
will normally make use of your operating system's
command processor, also known as the shell.
In Windows, the shell is usually the program named cmd.exe, although later versions of Windows come with a more powerful shell program called Powershell.
In Linux and on the Mac, the most popular shell program is bash (the Bourne-again Shell; a typical UNIX pun). That is the one we are using in our workspaces.
The Command Line
The command processor accepts an instruction (in text form),
interprets it, and then converts the request into the internal system calls needed to carry out the action. Shell commands list the files
in a directory or copy, move
and delete files.
The commands are often different for different shells. In Windows, you list files in the directory with the dir command, while in Linux and on the Mac, you use the ls command.
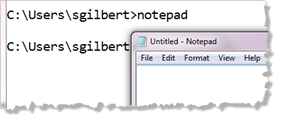
The most common shell command is to launch a program. Simply to type the name of the program into the shell, at the command prompt, like the picture shown here.
On Windows, when you type this and press ENTER the command-processor first searches a special area of memory (called the executable path), looking for an executable program that matches this pattern. When it finds one (notepad.exe) it launches the program.
If it cannot find the program (because it has not been added to the
executable path or because it hasn't been installed on the machine you are running
on), the command processor prints an error message. Here is one on Windows and
here is one on Unix.
On Windows, the current working directory is always placed in the executable path; on Linux and the Mac it is not; on those platforms, you have to type the current directory (./) before the name of the executable to launch it.
Command Line Arguments
Instead of prompting for input inside your program, you can supply additional information on the command line. These additional strings are called command line arguments.
Consider the make program. If you type this:
the make program receives "hello" as a command line argument. It is up to the program to decide what to do with it; make attempts to build a target named hello.
Strings starting with a hyphen (-) are customarily considered options (or switches) and other strings as file names. In Windows, such
switches often start with a forward slash. In Linux, when you type
ls
-la and press ENTER, you will get a similar display as if
you typed
dir
/ON
/X on Windows.
Using Command Line Arguments
To use command line arguments in your code, modify the signature of main() by adding two additional parameter variables: an integer (typically named argc), and an array of C-strings of type char*, typically named argv.
int main(int argc, char *argv[])
{
// your code here
}
The first parameter, (argc), is the number of elements in the command-line array. The second, (argv), is an array of char*, because each argument is passed as a character array or C-style string.
The first element, argv[0] is the name of the program, as invoked on the command line. A common error is to grab argv[0] when you really wanted the first argument, which is argv[1]. You need not use the names argc and argv, but it may confuse people if you don’t.
Traversing the Arguments
This program prints out the command-line by stepping through the array.
#include <iostream>
using namespace std;
int main(int argc, char *argv[])
{
cout << "The program name is " << argv[0] << endl;
for (int i = 1; i < argc; ++i)
{
cout << "Argument " << i << " is " << argv[i] << endl;
}
return 0;
}
Each whitespace-delimited cluster of characters on the command line is turned into a separate array argument. However, the rules for deciding what makes up a "cluster" are up to the operating system. Placing quotes around a pair of words works in any operating system; other features, like back-ticks, work only under some. Try some.
Converting Arguments
The command-line consists of C-style strings; to use any C++ string functions from the standard library, first assign the array element (argv[i]) to a local string variable. Once you've converted the C-string argument to a C++ string, you may then treat the argument as a number by converting it with the stoi(), stol() and stod() function in the <string> header.
However, you may convert directly from C-style strings to numbers, with out first converting to string, by using the helper functions in <cstdlib> which include atoi(), atol(), and atof().
#include <iostream>
#include <cstdlib>
using namespace std;
int main(int argc, char *argv[])
{
for (int i = 1; i < argc; ++i)
cout << atoi(argv[i]) << endl;
}
Notice that loop starts at 1 to skip over the program name at argv[0]. If you pass a floating-point number on the command line, atoi() takes only the digits up to the decimal point. If you pass non-numbers, these come back from atoi() as zero.