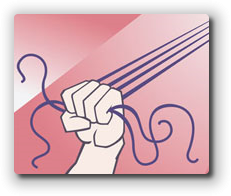
There are several different ways you to create stringobjects:
string s1; // empty string
string s2 lbrace;"Hello"};// explicitly initialized
string s3 = "World"; // Legacy C/Java style
string s4 lbrace;s3}; // a copy of s3
string s5 lbrace;'c', 'a', 't'}; // a sequence of chars
string s6 lbrace;R"("bob")"}; // a raw string
string s7(20, '-'); // 20 dashes
Let's look the most useful ones.
- In Java, s1 is a null string. (That is, it a String variable which contains the special value null, which cannot be used. Unlike Java, in C++, it is the empty string.
- s2 explicitly converts a string literal (character array) to a C++ string object. String literals, such as "hello" are not string objects, as they are in Java. Instead, they are pointers to a single character at the beginning of the literal.
- s3, the syntax you are probably most comfortable with, implicitly converts a C-string literal to a C++ string object.
- Produces a string that is a copy of the string s3.
- A string initialized with a sequence of char literals.
- Produces a string object from a raw string literal. Raw string literals begin with R"( and end with )". Inside you may store any character without using escape sequences.
- Produces a string made of 20 '-' characters. Note that char literals use single quotes, just as they do in Java. Python does not use the char type. Note that you must use parentheses for this constructor, not braces.
The {} and the () may often be used interchangably. However, for s5, you must use the braces {}, and for s7 you must use parentheses (). In C++98, you must use parentheses, not braces, and s5 and s6 will not work at all. These constructors, and raw strings were not added until C++11.
C++14 added C++ string literals, which is a regular C-string literal, with an s suffix, like "hello"s. This is no longer a pointer, but a full-fledged C++ string object, as in Java.
You may use >> and << to read and write string objects, like this:
cout << "Enter your name: ";
string name;
cin >> name;
cout << "Hello, " << name << "!" << endl;
This version of the program reads a string input by the user into the
variable name and then includes
name as part of the greeting, as shown in the screenshots below:
- If the user enters only a first name, then all goes as you'd expect.
-
However, the user enters a full name instead of just the first,
only the first is read.
Even though the program contains no code to split the name apart, it somehow still uses only the first name when it prints its greeting.
Why? Because >> stops reading as soon as it sees the first whitespace character. A whitespace character is any character that appears as blank space on the screen, and includes the tab and newline characters.
-
To read an entire line of text, use the
string function getline() like this, in place of line 3:
getline(cin, name);
This reads an entire line from cin into the variable name. When run, the program allows you to display the full name of the user instead of just the first name.
The <string> library redefines several standard operators using a C++ feature called operator overloading. When you use the + operator with numbers, it means addition, but, when you use it with the string type, it means concatenation.
string s1 = "hello", s2 = "world";
string s2 = s1 + " " + s2; // "hello world"
The shorthand += assignment operator has also been overloaded. It concatenates new text to the end of an existing string. You may concatenate char values to a string object, but you cannot concatenate numbers to string objectss as you could in Java.
string s{"abc"}; // uniform initialization
s += s; // ok, "abcabc"
s += "def"; // literal ok, "abcabcdef"
s += 'g'; // char ok, "abcabcdefg"
s = s + 2; // ERROR; no conversion
You cannot concatenate two string literals: "a" + "b" is illegal. However, separating them with whitespace, like "a" "b", is legal. Use this is used to join long lines together.
Comparisons
C++ overloads the relational operators so that you can compare string values just like primitive types. To see if the value of str is equal to "quit", just write this:
if (str == "quit") . . .
There is no need to use equals() or compareTo() as in Java.
Strings are compared using lexicographic ordering. Informally that means a string is smaller if it would appear earlier in the dictionary. However, when doing comparisons, case is significant, so "abc" is not equal to "ABC". Upper-case characters are "smaller" than lower-case characters, because they have smaller ASCII values.
In C++ string objects are mutable; you may change the individual characters inside a string variable. Compare this with Java or Python, where string objects are immutable.
string str = "hello";
str[0] = 'j';
cout << str << endl; // prints jello
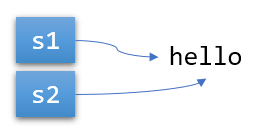
In Java and in Python, assignment of object types means that the variables are copied, but that the objects are not. Here' a piece of Java code which creates a String s1 and then creates a second, s2 initialized with s1. The illustration shows what this looks like in memory.
String s1 = "hello";
String s2 = s1;

C++ works differently. In Java and Python, variables refer to objects; in C++ variables contain objects. In C++, assigning one string to another, copies the underlying characters into an entirely new string, in the same way that assigning one int variable to another creates a new, independent variable and value.
Languages (like C++) that work like this have value semantics. In C++, the statementstr1 = str2
overwrites any previous contents of str1 with a copy of the characters contained in str2. The variables str1 and str2 therefore remain independent, which means that changing the characters in str1 does not affect str2.
Because string is a library or class type, it also has methods, just like the Java String class has methods such as length(), toUpper() and charAt(). In C++ instead of calling these methods, we use the term member function instead. Let's look at the difference between a regular (or "free") function in C++, and a member function.
In the string class, you've already seen the getline() function. The prototype for getline() looks like this:
istream& getline(istream&> in, string& str);
The function has two parameters: the input stream to read from, and the string object to modify; it returns a reference to its input stream (which may be ignored).
string line;
getline(cin, line);
Although getline() is declared inside the <string> header, it is not part of the string class; it is just a regular function. Member functions, in contrast, are part of a class, and, as in Java, they are called by using a special syntax:
receiver.request(arguments);
In this case, receiver is an object, and request is a member function defined in that class. When compiled, the address of the receiver object is passed to the member function as an invisible or implicit first parameter. Inside the member function, that implicit parameter is accessed using the keyword this, in a manner similar to Java.