This is the Course Reader for CS 150, C++ Programming I, at Orange Coast College. CS 150 is a second-semester course for majors who have already taken a course covering variables, I/O, calculations, loops, decisions, functions and arrays.
The reader is short and concise, in line with Leonard Elmore's advice: leave out the parts readers skip. It focuses on the parts you need to get your work done.
To get more details, consult one of these highly recommended texts.
- The C++ Primer, 5th Edition. Lippman, Lajoie and Moo
- The C++ Programming Language, 4th Edition, Bjarne Stroustrup
These are professional books to keep on your shelf after the course is over. Throughout the text you'll also find links pointing to additional reading, which is optional.
C++ is a compiled, high-level programming language. Compilers produce native machine code programs, which run directly on your hardware, without an interpreter (like Python) or intermediate software, such as the Java Virtual Machine. Even though C++ programs are often more efficient than their Java or Python counterparts, certain kinds of errors cannot easily be detected, so you will find that you must be more careful and detail-oriented when writing programs in C++.
C++ is also a standardized language, specified by the ISO,
or International Standards Organization. Unlike proprietary languages, controlled by a single company, anyone may implement the C++ language without fear of lawsuits. There are several versions of C++ you should know about.- Pre-standard C++: 1980-98. Often incompatible versions, vendor specific.
- C++ 98: the first standard version; it was updated in 2003 ( C++03).
- C++ 11: the second major standard; it was updated in C++14 and C++ 17.
- C++20, the latest major standard, is now complete. Most recent compilers offer a sampling of some of its new features.
In this class we will be using C++ 17. Compilers that implement C++17 include Visual Studio 2019, GNU g++ 7 (or later), and clang 6+.
On the first page of Dennis Ritchie's seminal programming book, The C Programming Language, he writes:
The only way to learn a new programming language is by writing programs in it. The first program to write is the same for all languages:
Print the words: hello, world
This is the big hurdle; to leap over it you have to be able to create the program text somewhere, compile it successfully, load it, run it, and find out where the output went. With these mechanical details mastered, everything else is comparatively easy.
I'm sure his last sentence was somewhat
tongue-in-cheek, but let's follow his example and look at a C++ version of "Hello
World" by clicking the little "running-man" to your right to open the program
in an online IDE. Leave it open for the next few pages.
When you click the link you'll find the human-readable source code for this program, in the file which we would name hello.cpp.
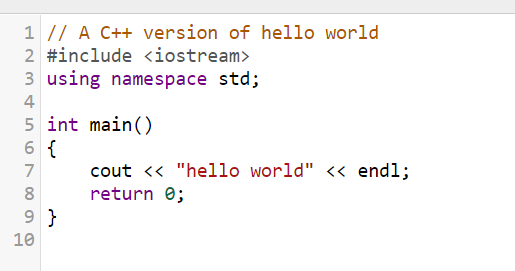
Let's review each line:
- A comment, designed for the reader, but ignored by the compiler.
- A preprocessor directive which makes the C++ library facilities available.
- A namespace directive makes standard names available without qualification.
- A blank line, used to make your code more readable.
-
The
main
function or entry point to your program. Each program has onemain
. - The opening brace. How you say begin the actions in C++.
-
Prints to the console, using the standard output object named
cout
. -
Ends the main function, returning the value
0
back to the operating system. -
The closing brace marks the end of
main
, matching the opening brace.
To create your own C++ programs, you follow a mechanical process, a well-defined set of steps called the edit-build-run cycle. In short you:
- Create your source code using a text editor.
- Build your executable using a compiler toolchain
- Run your program using your operating system facilities
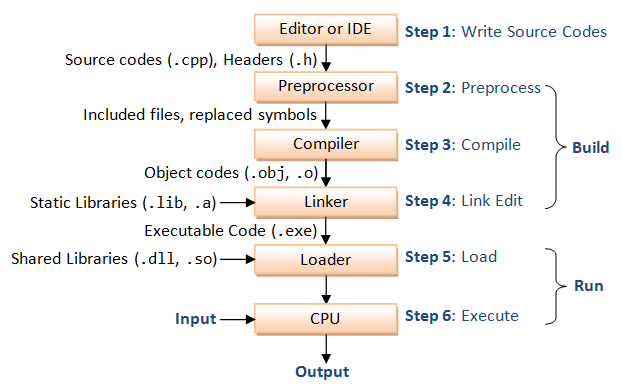
The Build Process
Once you have written your source code, the build process turns that source code into an executable program. The build process involves several tools:
- Preprocessor—performs text substitution on your source code.
- Compiler—generates assembly code from the preprocessed source code.
- Assembler—converts assembly code into object or native machine code.
- Linker—combines machine-code modules into an executable that runs.
- Make—provides instructions for building each program.
- Loader—reads the executable from disk into memory and starts it running.
- Debugger—runs your program inside a controlled environment.
A combination a C++ compiler, linker, assembler and libraries, is known as a toolchain. The toolchain we'll use for this course is the Linux GCC 9 toolchain. In an online IDE, like the one in these lessons, you just click the Run button to perform all the steps and run the executable. In lecture, you'll look at each of these steps in more detail.